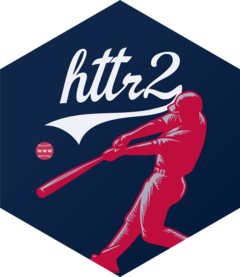
Package index
-
request()
- Create a new HTTP request
-
req_body_raw()
req_body_file()
req_body_json()
req_body_json_modify()
req_body_form()
req_body_multipart()
- Send data in request body
-
req_cookie_preserve()
- Preserve cookies across requests
-
req_headers()
- Modify request headers
-
req_method()
- Set HTTP method in request
-
req_options()
- Set arbitrary curl options in request
-
req_progress()
- Add a progress bar to long downloads or uploads
-
req_proxy()
- Use a proxy for a request
-
req_template()
- Set request method/path from a template
-
req_timeout()
- Set time limit for a request
-
req_url()
req_url_query()
req_url_path()
req_url_path_append()
- Modify request URL
-
req_user_agent()
- Set user-agent for a request
-
last_response()
last_request()
- Retrieve most recent request/response
-
req_dry_run()
- Perform a dry run
-
req_verbose()
- Show extra output when request is performed
-
with_mocked_responses()
local_mocked_responses()
- Temporarily mock requests
-
with_verbosity()
- Temporarily set verbosity for all requests
-
req_auth_basic()
- Authenticate request with HTTP basic authentication
-
req_auth_bearer_token()
- Authenticate request with bearer token
-
req_oauth_auth_code()
oauth_flow_auth_code()
- OAuth with authorization code
-
req_oauth_bearer_jwt()
oauth_flow_bearer_jwt()
- OAuth with a bearer JWT (JSON web token)
-
req_oauth_client_credentials()
oauth_flow_client_credentials()
- OAuth with client credentials
-
req_oauth_device()
oauth_flow_device()
- OAuth with device flow
-
req_oauth_password()
oauth_flow_password()
- OAuth with username and password
-
req_oauth_refresh()
oauth_flow_refresh()
- OAuth with a refresh token
-
req_perform()
- Perform a request to get a response
-
req_perform_stream()
- Perform a request and handle data as it streams back
Control the process
These functions don’t modify the HTTP request that is sent to the server, but affect the overall process of req_perform()
.
-
req_cache()
- Automatically cache requests
-
req_error()
- Control handling of HTTP errors
-
req_throttle()
- Rate limit a request by automatically adding a delay
-
req_retry()
- Control when a request will retry, and how long it will wait between tries
-
req_perform_iterative()
experimental - Perform requests iteratively, generating new requests from previous responses
-
req_perform_parallel()
- Perform a list of requests in parallel
-
req_perform_sequential()
- Perform multiple requests in sequence
-
iterate_with_offset()
iterate_with_cursor()
iterate_with_link_url()
- Iteration helpers
-
resps_successes()
resps_failures()
resps_requests()
resps_data()
- Tools for working with lists of responses
-
resp_body_raw()
resp_has_body()
resp_body_string()
resp_body_json()
resp_body_html()
resp_body_xml()
- Extract body from response
-
resp_check_content_type()
- Check the content type of a response
-
resp_content_type()
resp_encoding()
- Extract response content type and encoding
-
resp_date()
- Extract request date from response
-
resp_headers()
resp_header()
resp_header_exists()
- Extract headers from a response
-
resp_link_url()
- Parse link URL from a response
-
resp_raw()
- Show the raw response
-
resp_retry_after()
- Extract wait time from a response
-
resp_status()
resp_status_desc()
resp_is_error()
resp_check_status()
- Extract HTTP status from response
-
resp_url()
resp_url_path()
resp_url_query()
resp_url_queries()
- Get URL/components from the response
-
curl_translate()
curl_help()
- Translate curl syntax to httr2
-
secret_make_key()
secret_encrypt()
secret_decrypt()
secret_write_rds()
secret_read_rds()
secret_decrypt_file()
secret_encrypt_file()
secret_has_key()
- Secret management
-
obfuscate()
obfuscated()
- Obfuscate mildly secret information
-
url_parse()
url_build()
- Parse and build URLs
-
oauth_cache_path()
- httr2 OAuth cache location
-
oauth_client()
- Create an OAuth client
-
oauth_client_req_auth()
oauth_client_req_auth_header()
oauth_client_req_auth_body()
oauth_client_req_auth_jwt_sig()
- OAuth client authentication
-
oauth_redirect_uri()
- Default redirect url for OAuth
-
oauth_token()
- Create an OAuth token