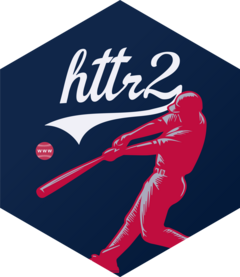
Perform request asynchronously using the promises package
Source:R/req-promise.R
req_perform_promise.Rd
This variation on req_perform()
returns a promises::promise()
object immediately
and then performs the request in the background, returning program control before the request
is finished. See the
promises package documentation
for more details on how to work with the resulting promise object.
If using together with later::with_temp_loop()
or other private event loops,
a new curl pool made by curl::new_pool()
should be created for requests made
within the loop to ensure that only these requests are being polled by the loop.
Like with req_perform_parallel()
, exercise caution when using this function;
it's easy to pummel a server with many simultaneous requests. Also, not all servers
can handle more than 1 request at a time, so the responses may still return
sequentially.
req_perform_promise()
also has similar limitations to the
req_perform_parallel()
function, it:
Will not retrieve a new OAuth token if it expires after the promised request is created but before it is actually requested.
Does not perform throttling with
req_throttle()
.Does not attempt retries as described by
req_retry()
.Only consults the cache set by
req_cache()
when the request is promised.
Arguments
- req
A httr2 request object.
- path
Optionally, path to save body of the response. This is useful for large responses since it avoids storing the response in memory.
- pool
Optionally, a curl pool made by
curl::new_pool()
. Supply this if you want to override the defaults for total concurrent connections (100) or concurrent connections per host (6).
Value
a promises::promise()
object which resolves to a response if
successful or rejects on the same errors thrown by req_perform()
.
Examples
if (FALSE) { # \dontrun{
library(promises)
request_base <- request(example_url()) |> req_url_path_append("delay")
p <- request_base |> req_url_path_append(2) |> req_perform_promise()
# A promise object, not particularly useful on its own
p
# Use promise chaining functions to access results
p %...>%
resp_body_json() %...>%
print()
# Can run two requests at the same time
p1 <- request_base |> req_url_path_append(2) |> req_perform_promise()
p2 <- request_base |> req_url_path_append(1) |> req_perform_promise()
p1 %...>%
resp_url_path %...>%
paste0(., " finished") %...>%
print()
p2 %...>%
resp_url_path %...>%
paste0(., " finished") %...>%
print()
# See the [promises package documentation](https://rstudio.github.io/promises/)
# for more information on working with promises
} # }